Container image
How to build a container image
In this part, we will build the container image, using a Dockerfile.
A Dockerfile is a text file with no extension, containing specifications for a software executable field, and structure for Docker Image. From those commands, Docker will build a Docker image (usually from a few MB to a few GB in size).
Dockerfile overview
The general syntax of a Dockerfile
- INSTRUCTION is the name of the directives contained in Dockerfile, each directive performing a certain task, specified by Docker.
- When declaring these directives, they must be written in CAPITAL.
- A Dockerfile must begin with the FROM directive to declare which image will be used as the background to build your image.
- arguments is the body of the directives, which decides what the directive will do.
Some directives in Dockerfile
FROM
FROM <image> [AS <name>]
FROM <image>[:<tag>] [AS <name>]
FROM <image>[@<digest>] [AS <name>]
LABEL
LABEL <key>=<value> <key>=<value> <key>=<value> ... <key>=<value>
MAINTAINER
MAINTAINER <name> [<email>]
RUN
RUN <command>
ADD
ADD [--chown=<user>:<group>] <src>... <dest>
ADD [--chown=<user>:<group>] ["<src>",... "<dest>"]
COPY
COPY [--chown=<user>:<group>] <src>... <dest>
COPY [--chown=<user>:<group>] ["<src>",... "<dest>"]
ENV
CMD Used to provide default command to be run when Docker Container starts from built Image, there can be only 1 CMD directive.
Practice
- Create a folder for container image
mkdir ~/environment/container-image
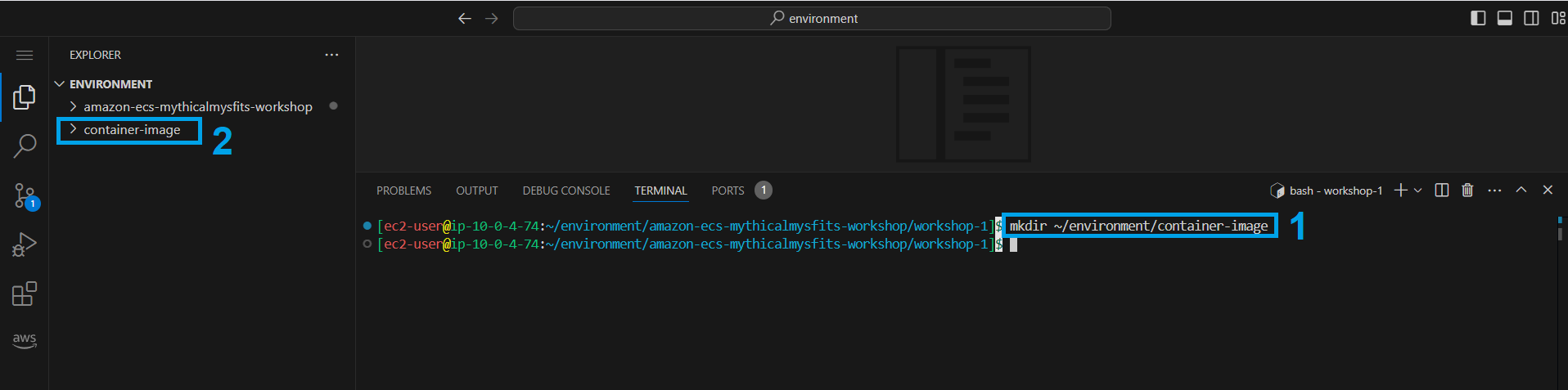
- Type cd container-image to change into that directory.
cd ~/environment/container-image
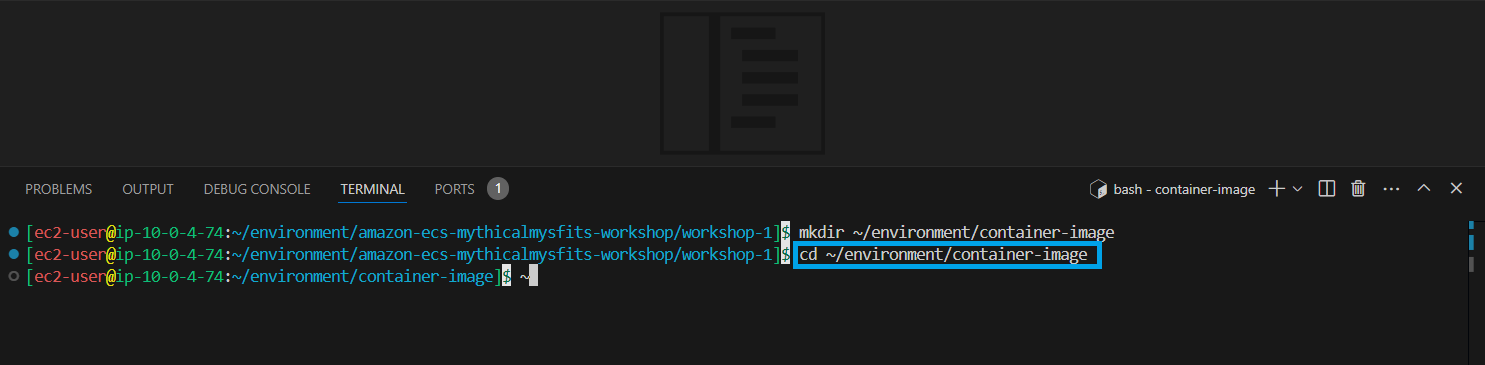
- Run touch Dockerfile to create the Dockerfile. This file will contain a set of steps needed to build the container image.
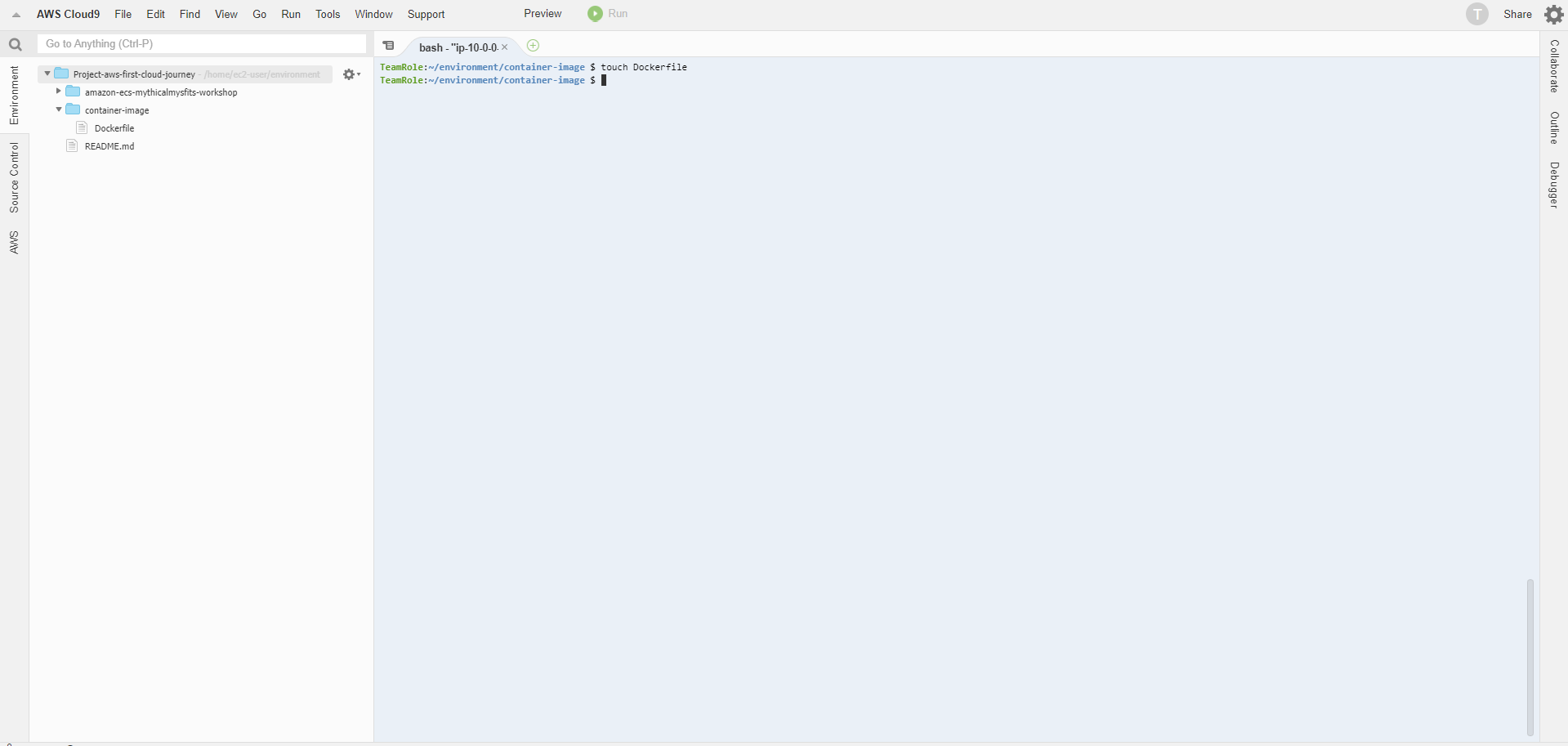
- Run below command to update Dockerfile content
cat <<EOF> Dockerfile
FROM nginx\:latest
COPY index.html /usr/share/nginx/html
EOF
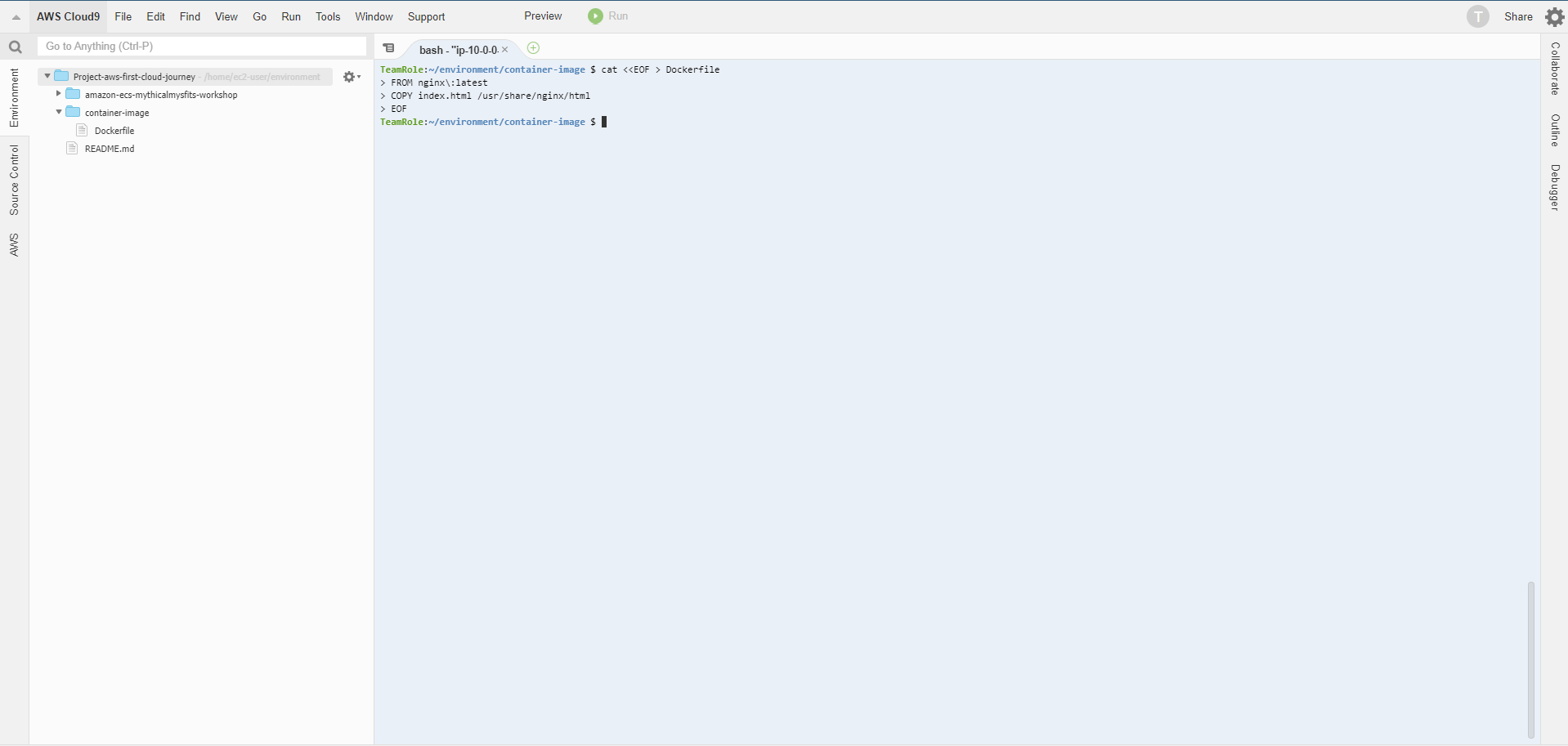
- Run touch index.html to create an empty html file that will contain a simple message.
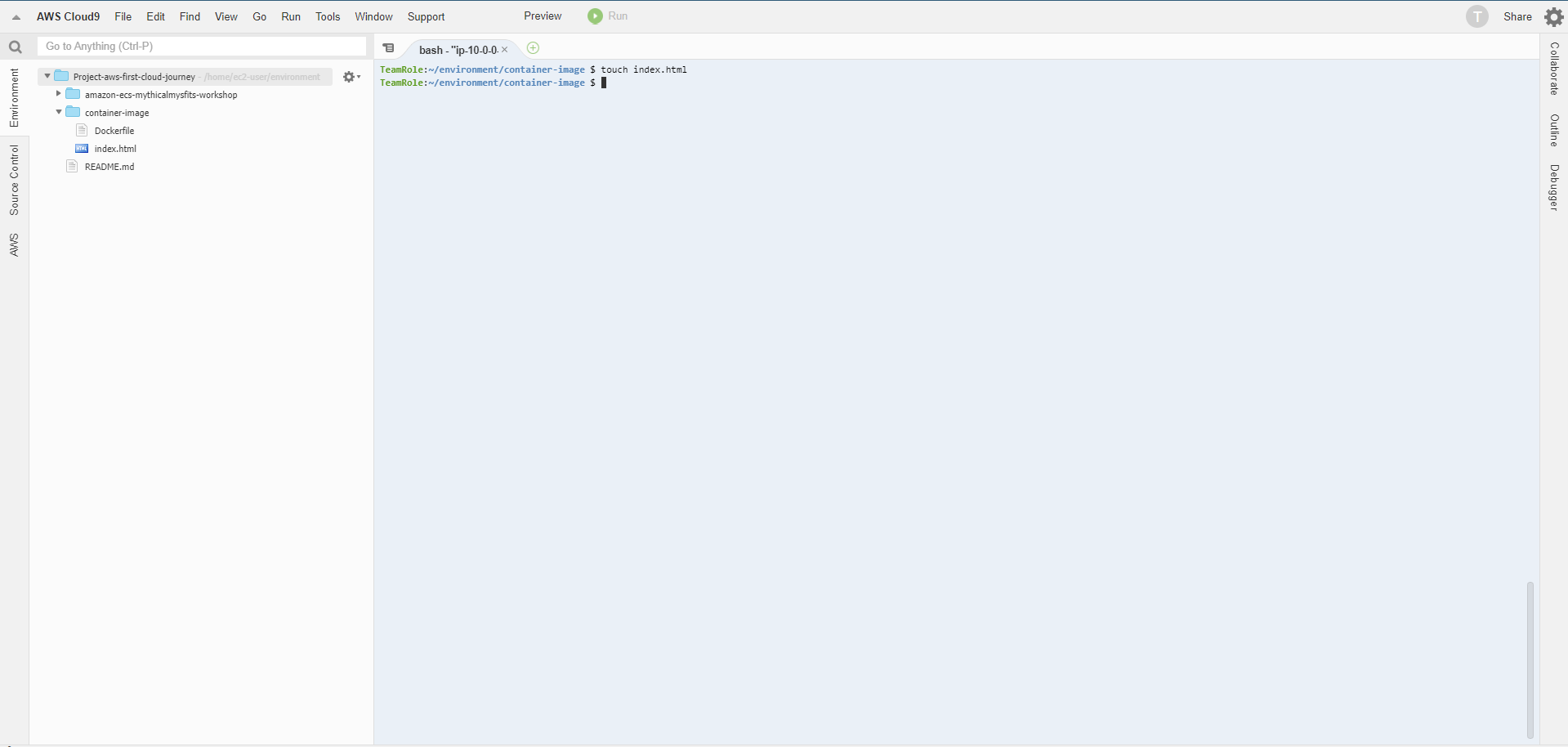
- Use echo to pass a simple message into the index.html file
echo "We've added our own custom content into the container" >> index.html
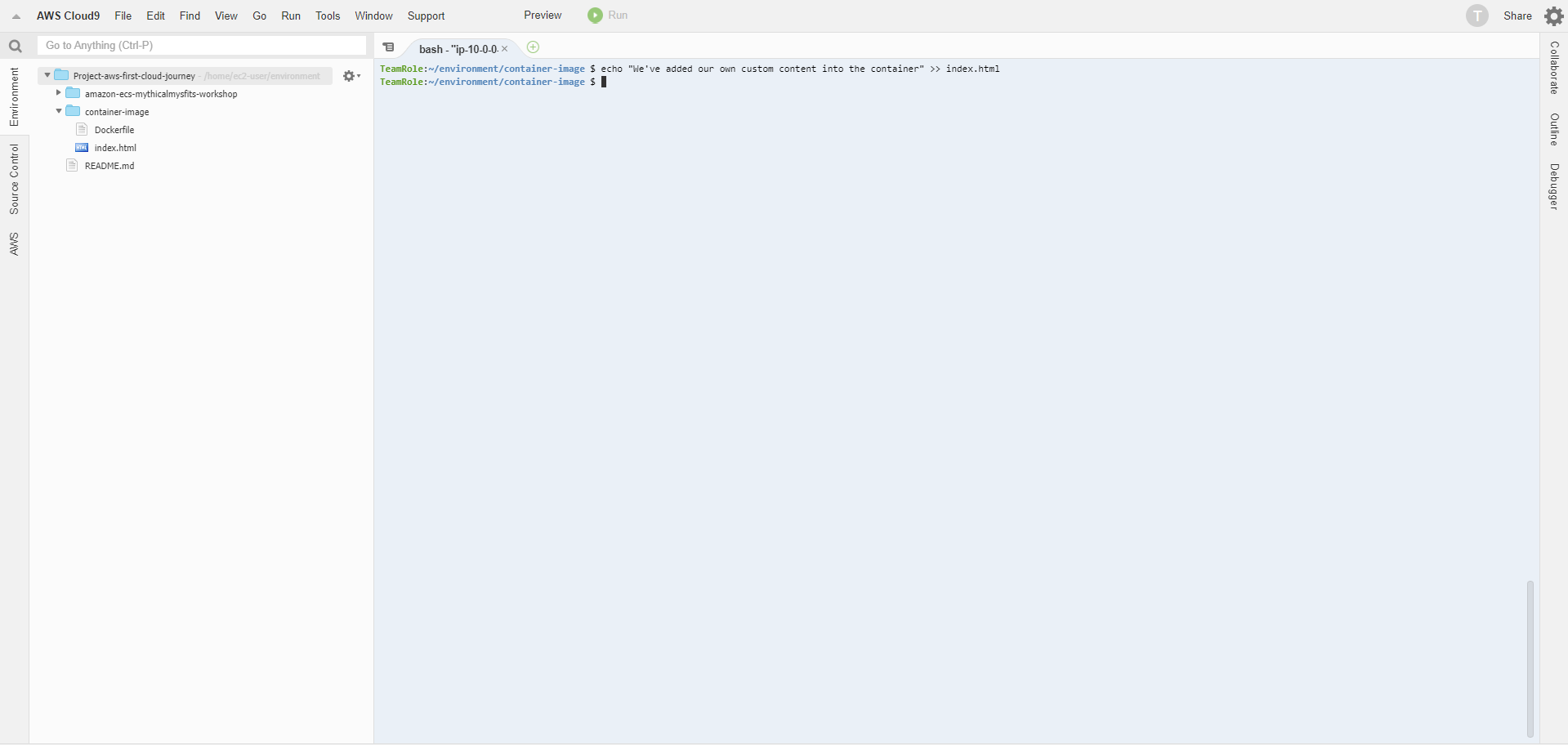
- Use docker build -t nginx:1.0 . to build the nginx container image from the Dockerfile.
docker build -t nginx:1.0 .
- Refer to the build image command from a Dockerfile
docker build [OPTIONS] PATH | URL | -
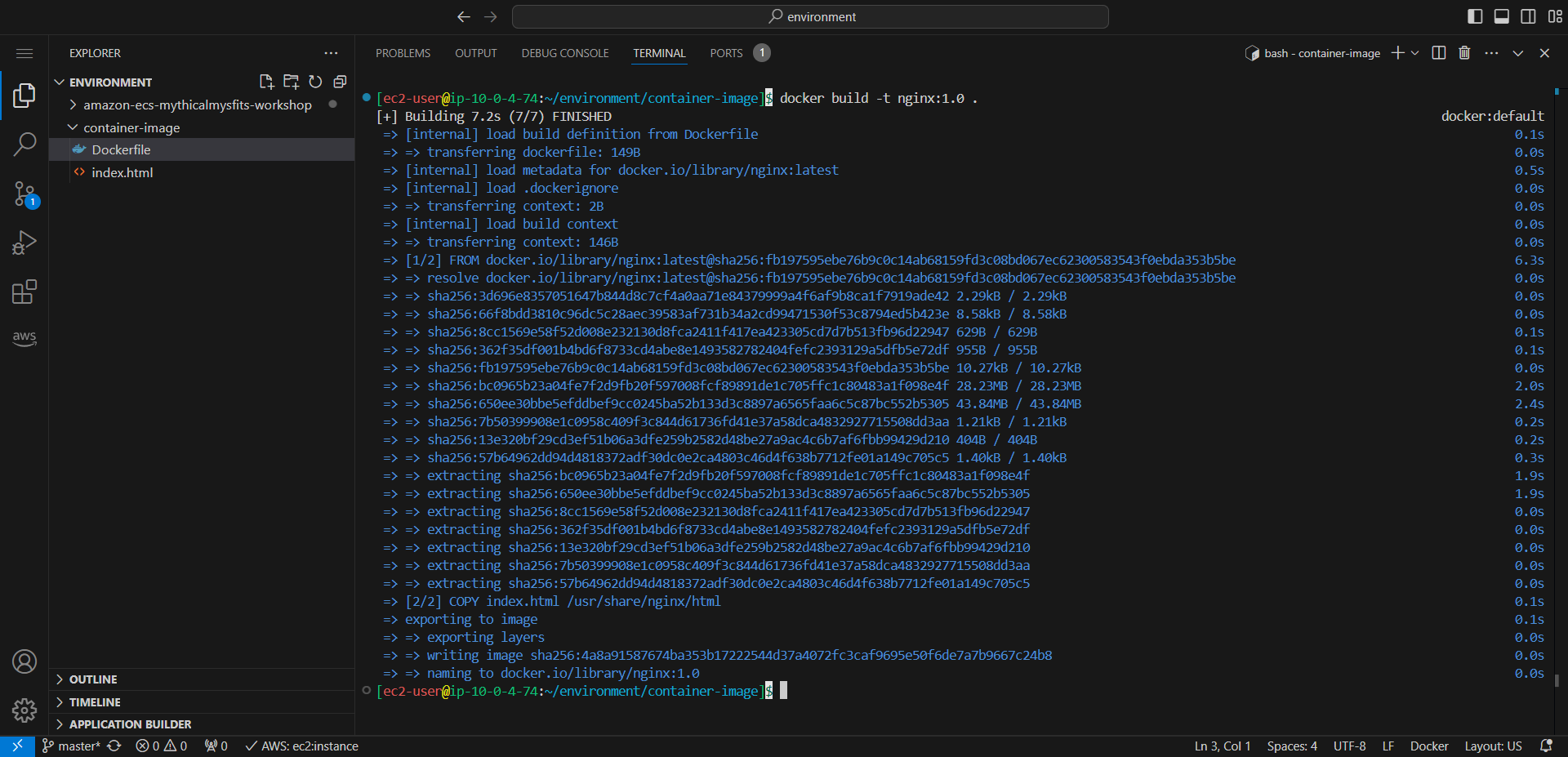
- Use docker history nginx:1.0 to see all steps and base container.
docker history nginx:1.0
- Refer to the command to view the history of an image:
docker history [OPTIONS] IMAGE
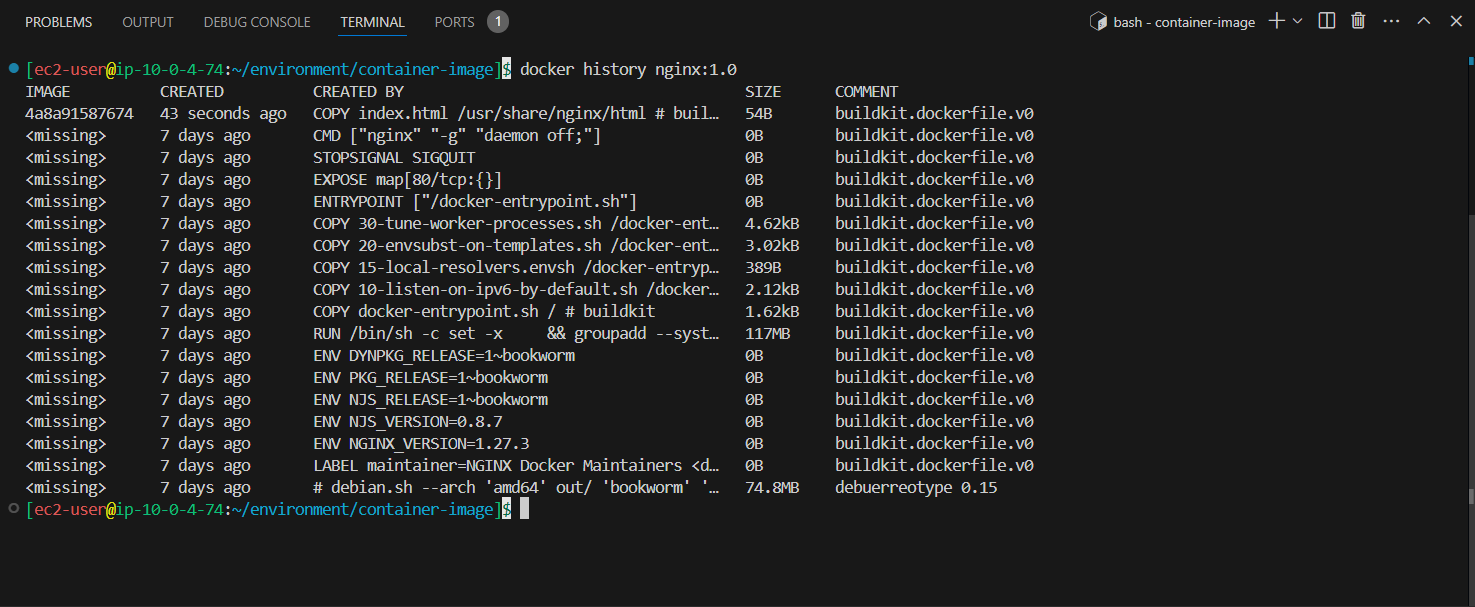
- Use the command docker run -p 8081:80 –name nginx nginx:1.0 to run the container (don’t use background mode for easy debugging)
docker run -p 8081:80 --name nginx nginx:1.0
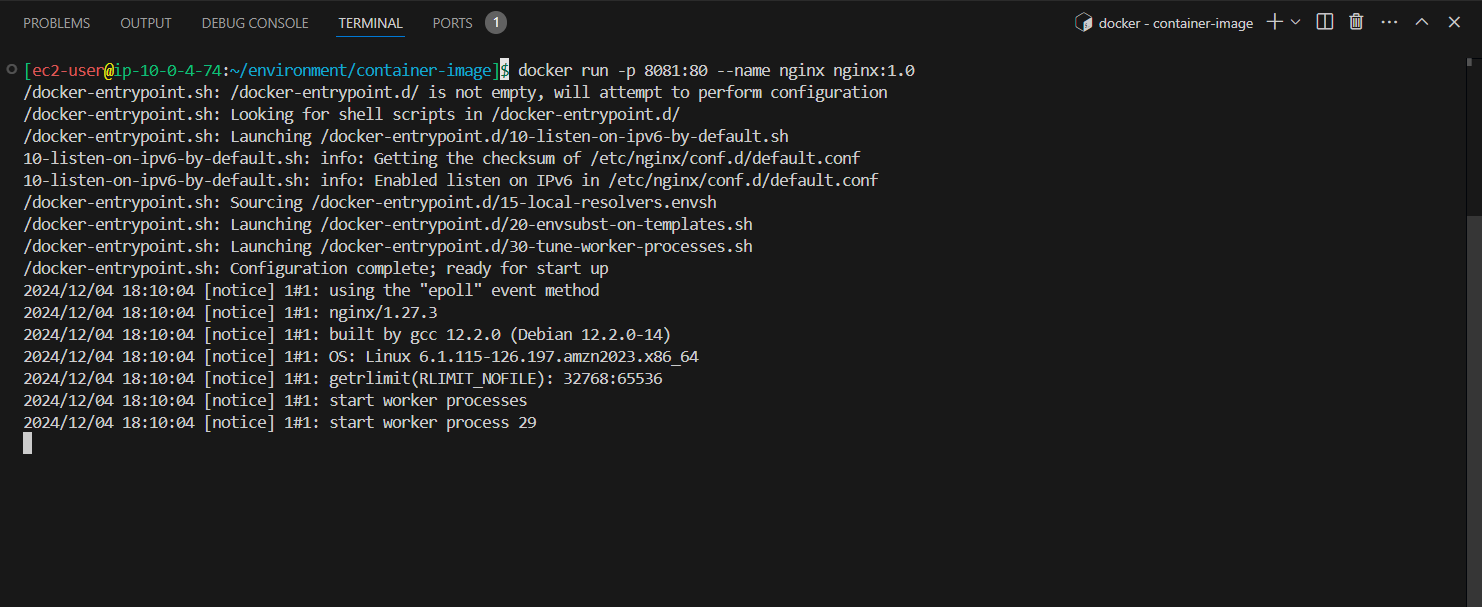
- Open another Terminal tab ( Window -> New Terminal ). Run curl http://localhost:8081 in the tab a few times and see what’s new.
curl http://localhost:8081

- Go back to the first tab and see the log lines sent immediately to STDOUT. Type Ctrl-C to exit the log output. Note that the container has been stopped but is still there when running docker ps -a.
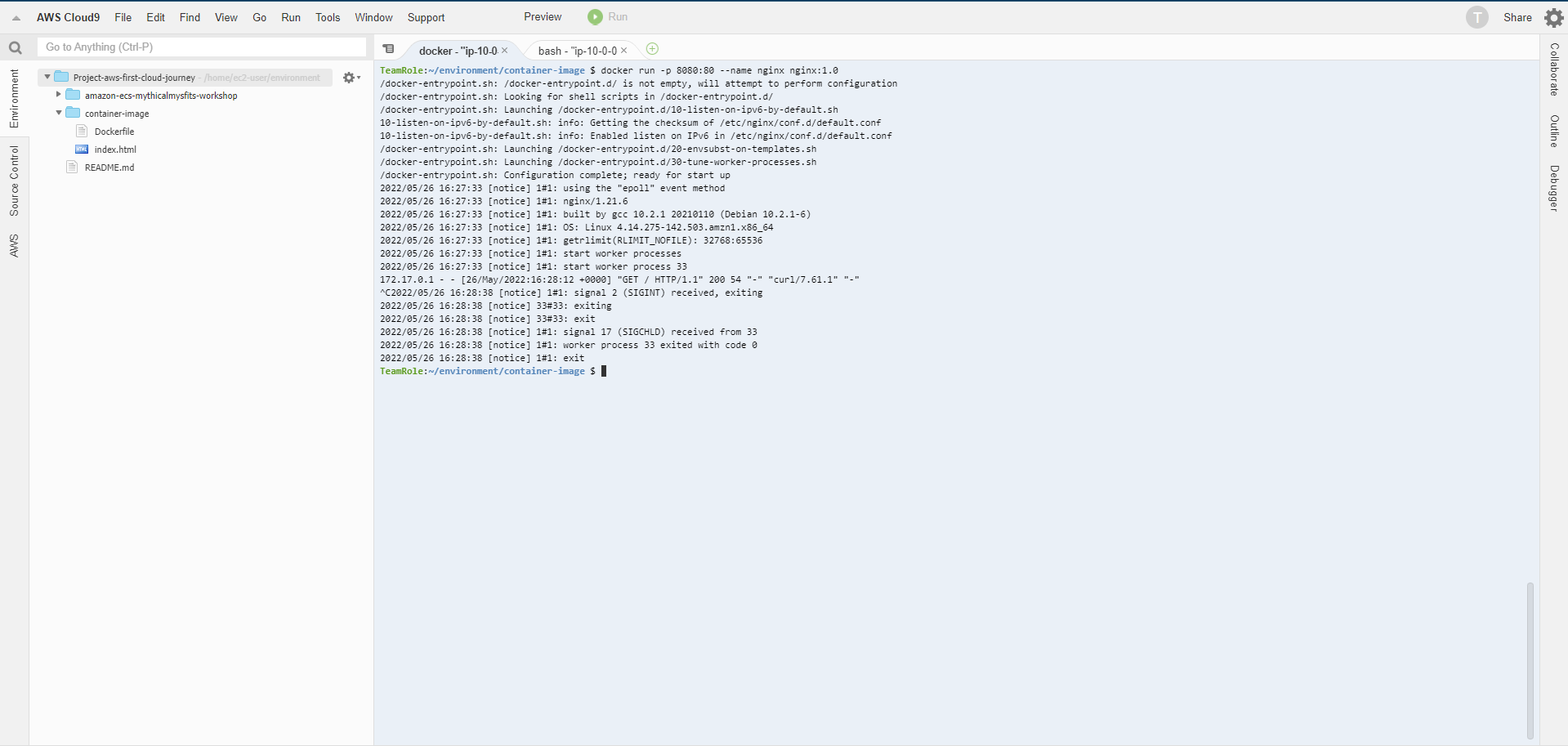
- Using docker ps -a

- Use sudo docker inspect nginx to see detailed information about stopped containers.
sudo docker inspect nginx
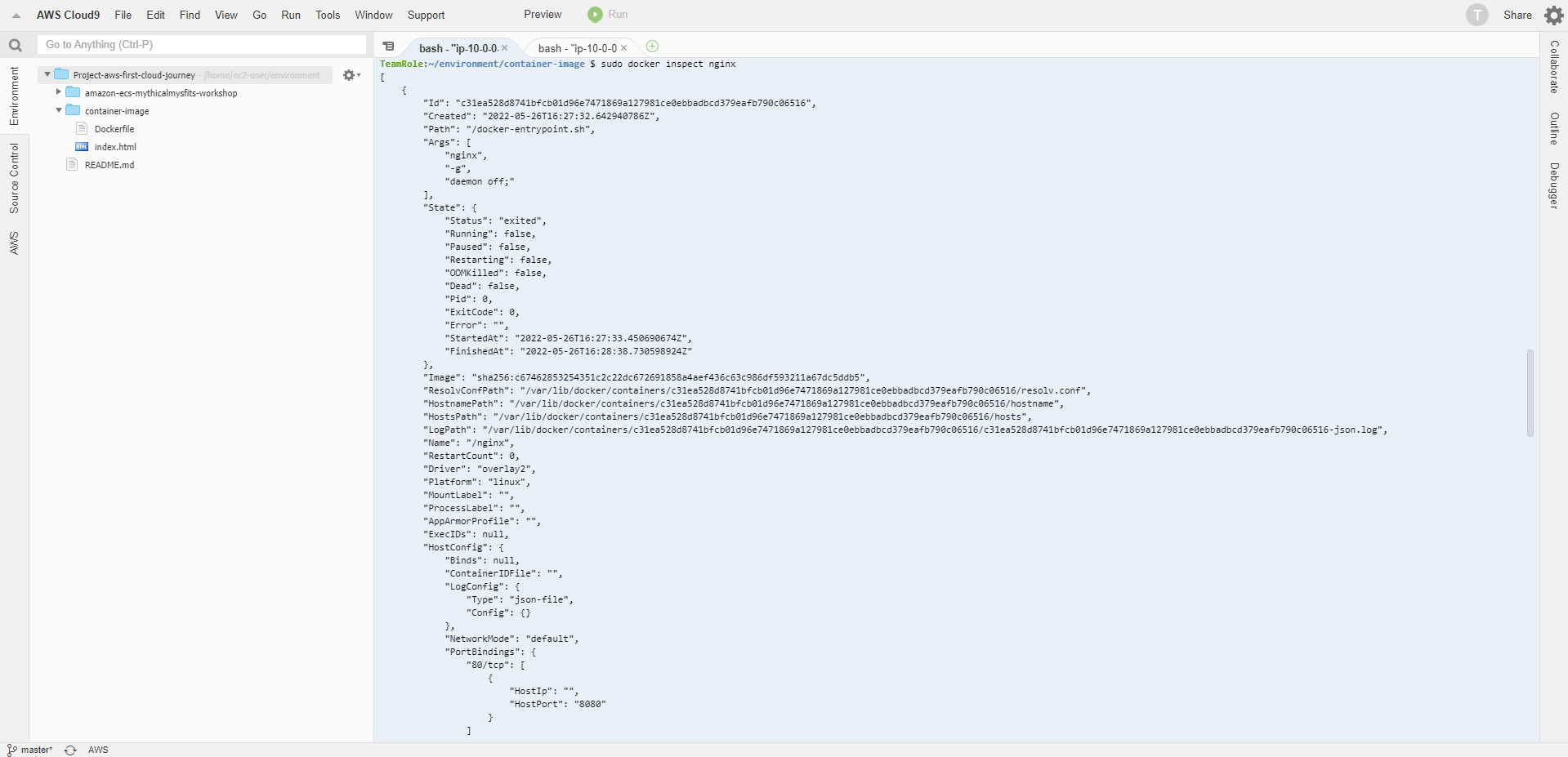
- Use docker rm nginx to delete the container
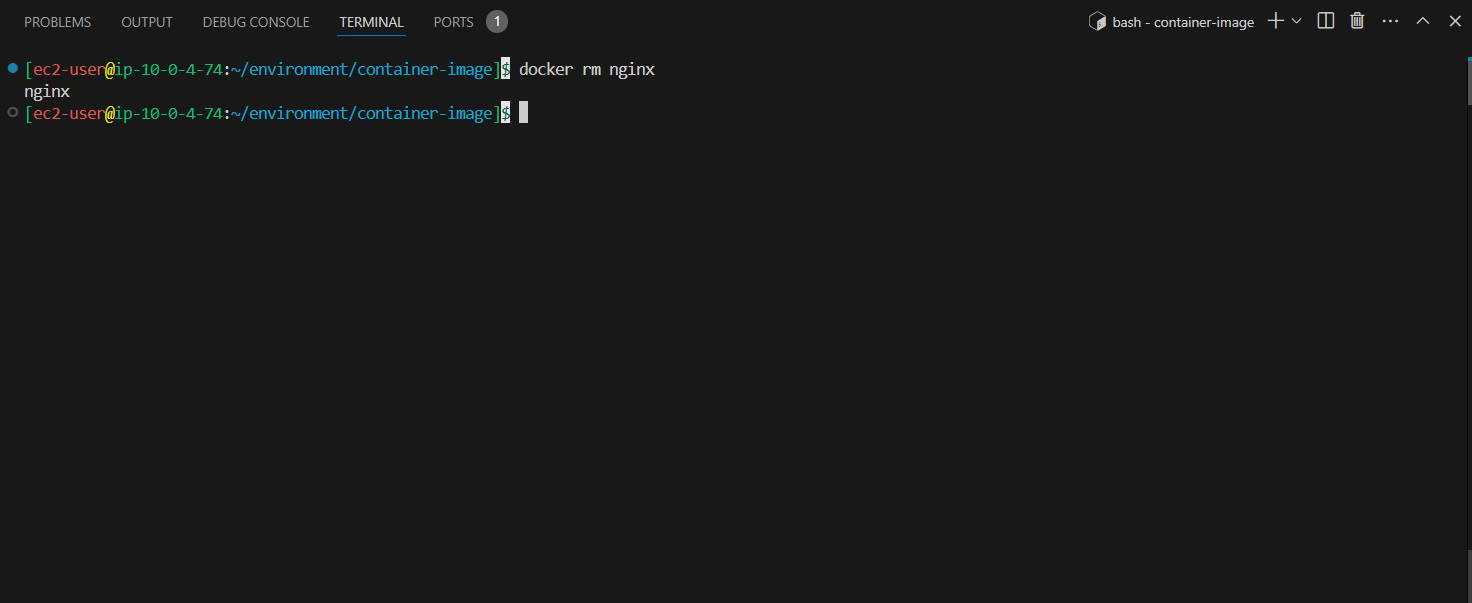
- Mount some files from the host into the container instead of embedding them in the image.
docker run -d -p 8081:80 -v /home/ec2-user/environment/container-image/index.html:/usr/share/nginx/html/index.html\:ro --name nginx nginx:latest
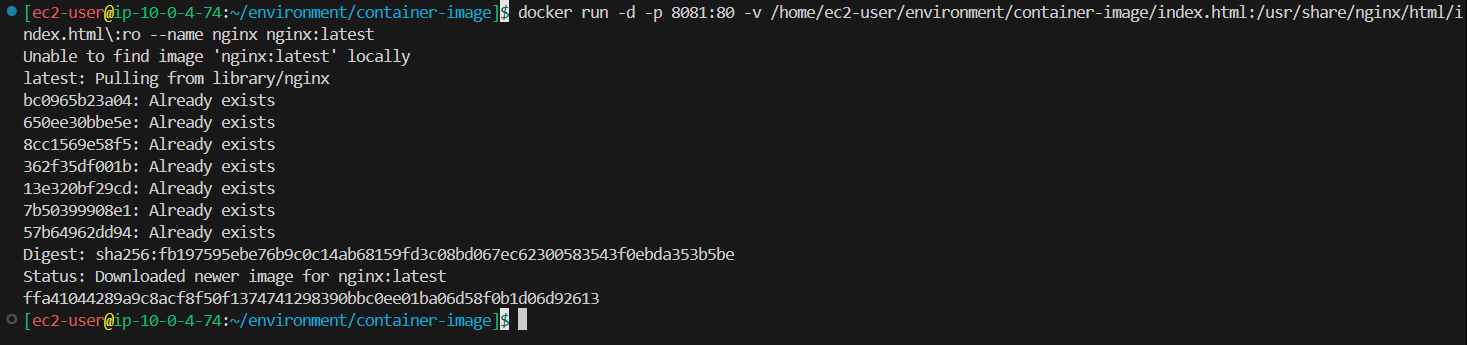
- Execute curl http://localhost:8081. Note that although this is an upstream nginx image from Docker Hub, the content is there.
curl http://localhost:8081
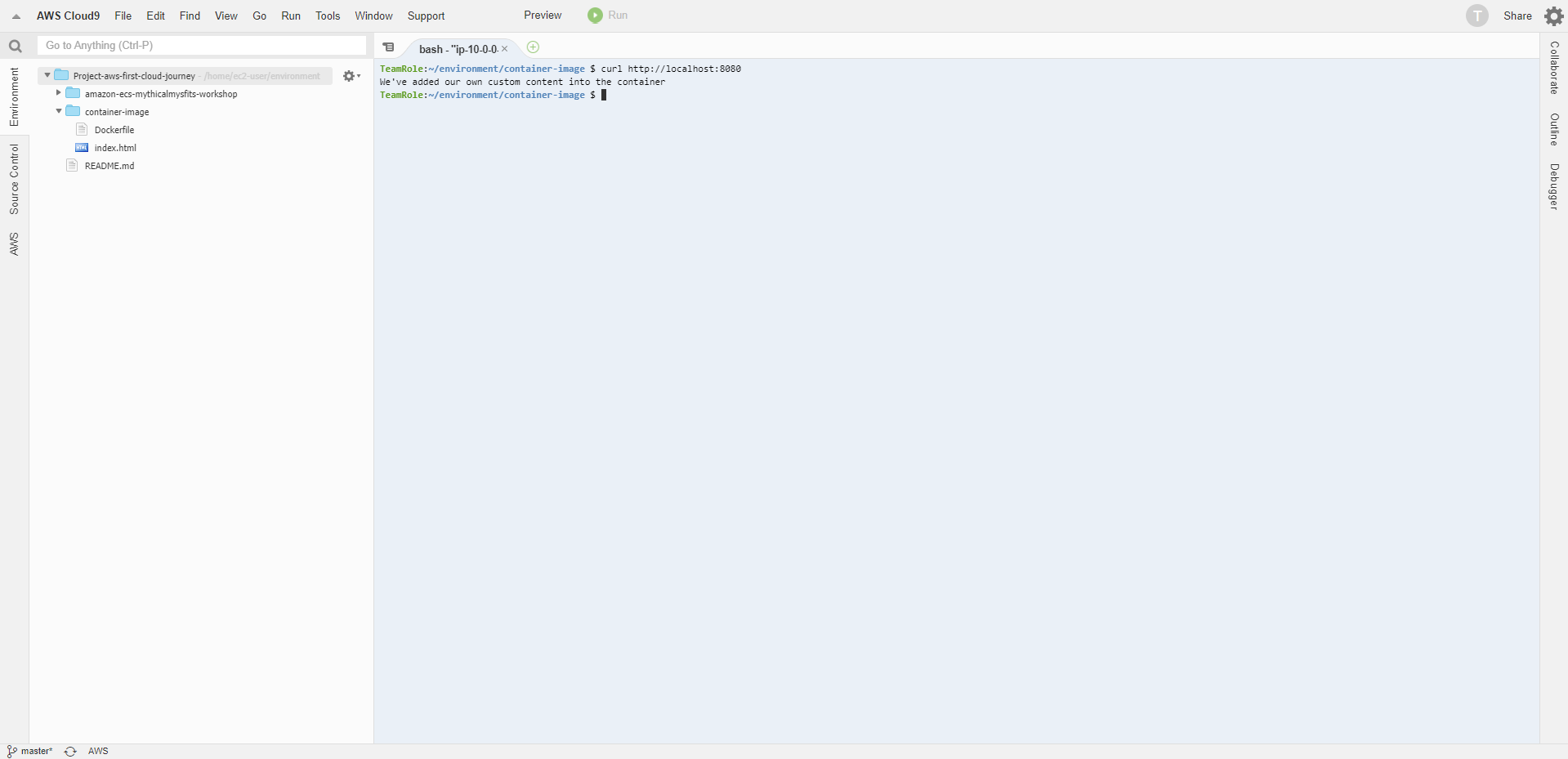
- Edit the index.html. file
echo "This is another line I've added to my container" >> index.html
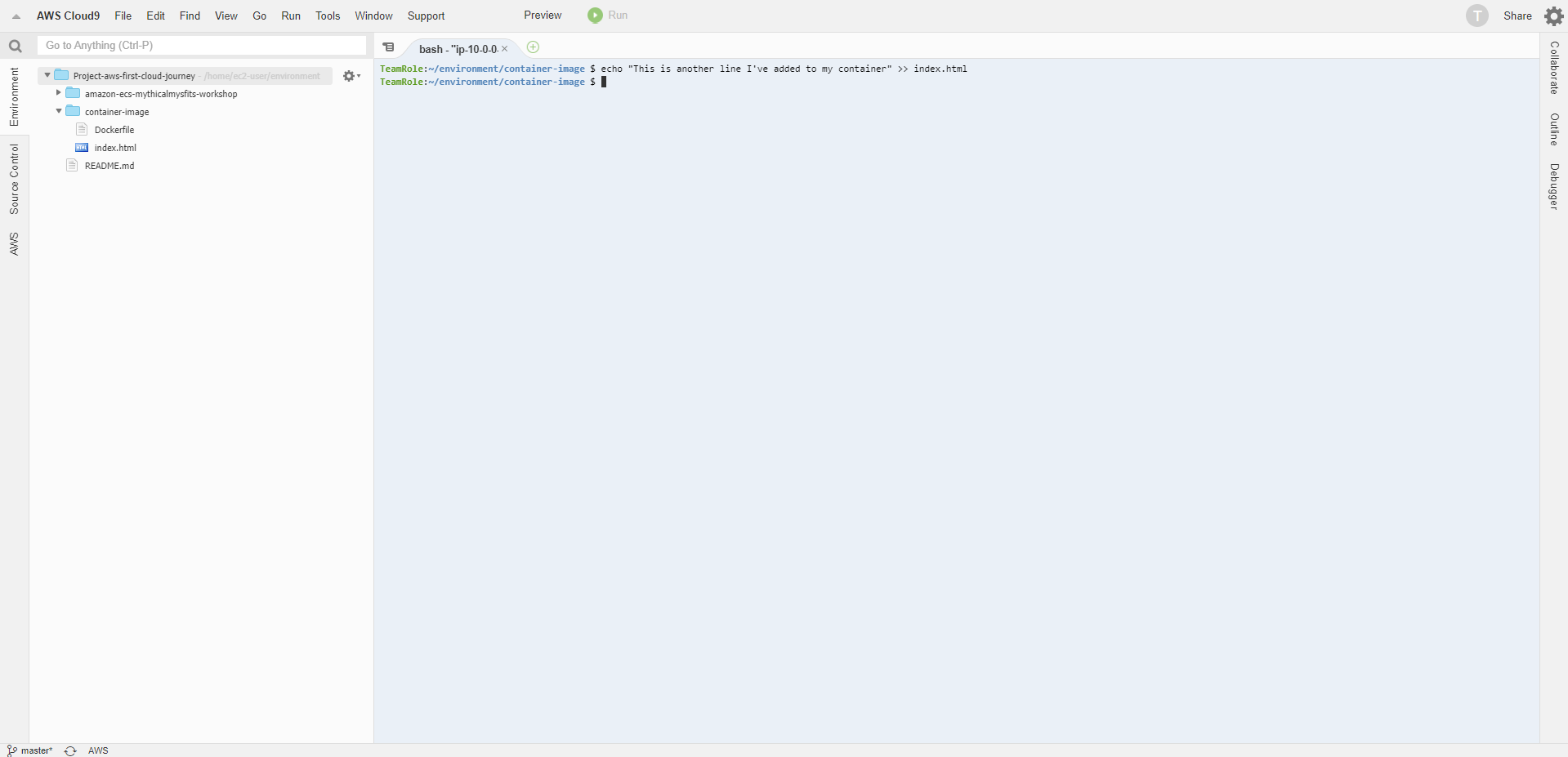
- Try again curl http://localhost:8081
curl http://localhost:8081
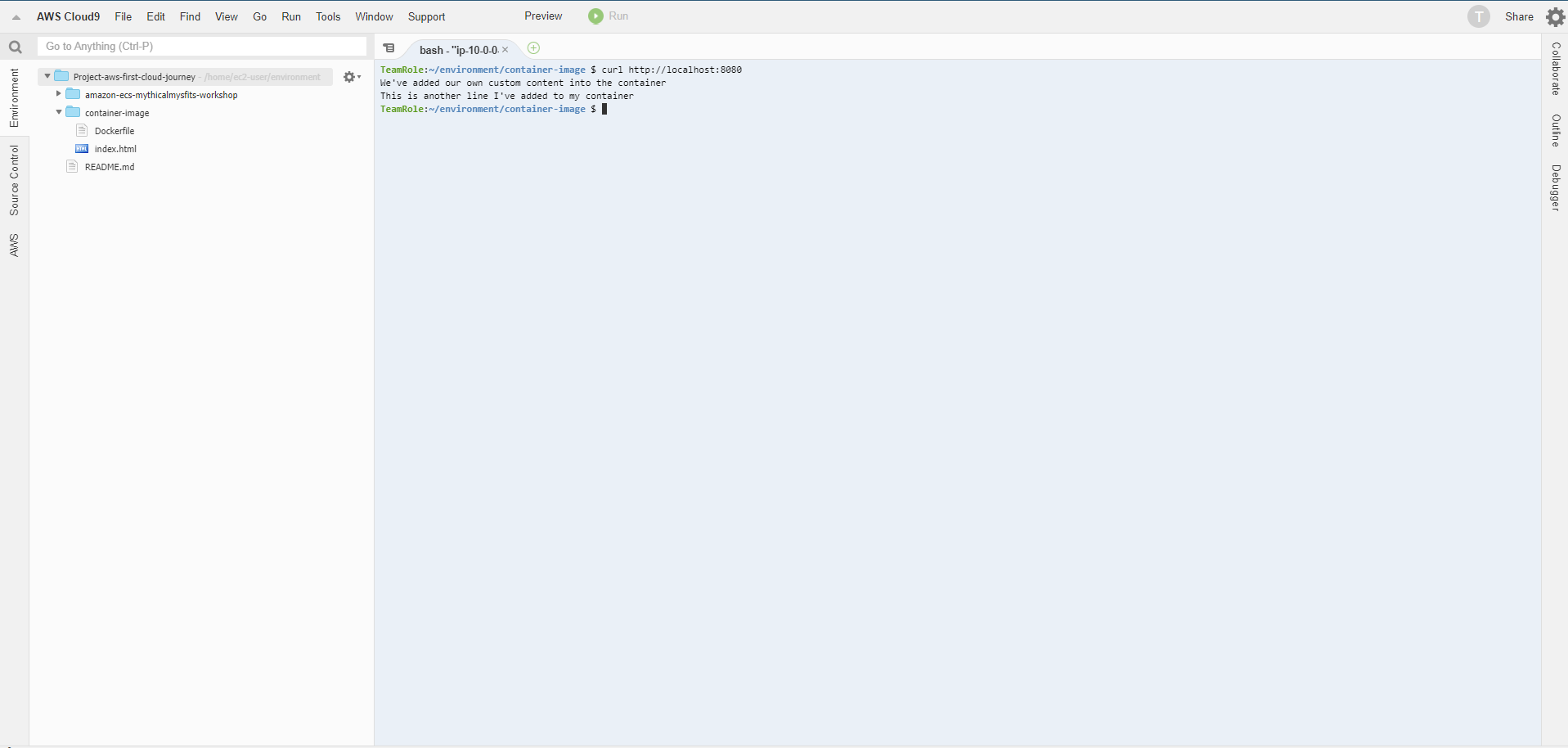
- Finally, run docker stop nginx and docker rm nginx to stop and remove the container
docker stop nginx && docker rm nginx
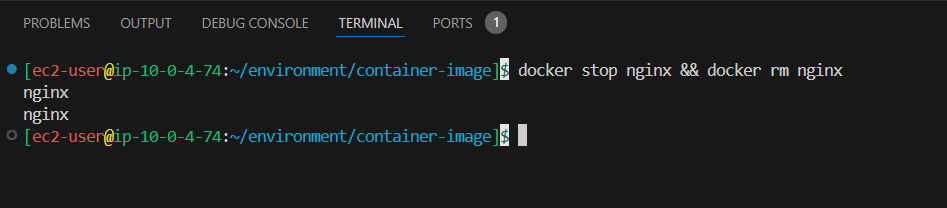